Here’s a cheat sheet with some of the most common JavaScript syntax and concepts:
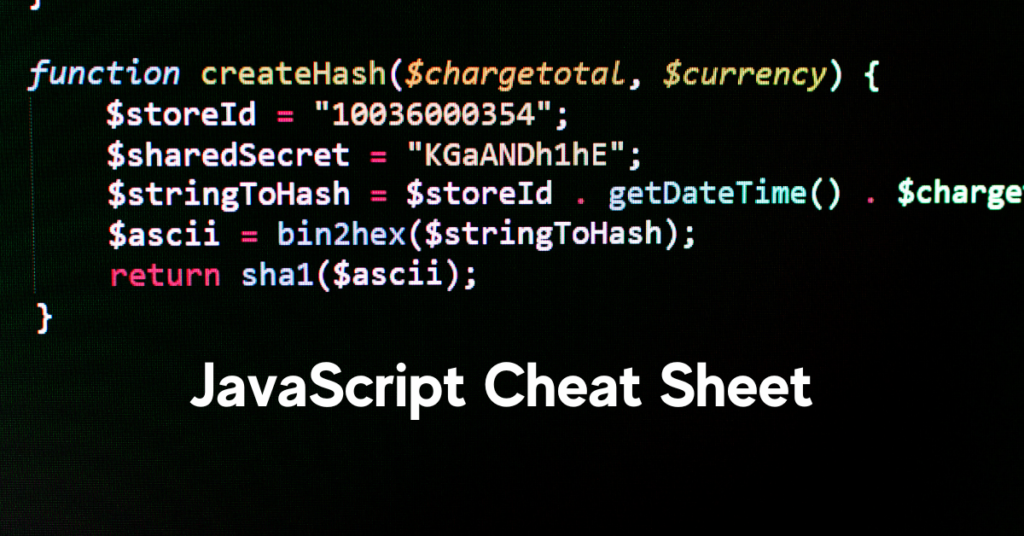
Variables and Data Types
Discover variable declaration, data types (strings, numbers, booleans), and how to scope variables efficiently.
// declaring variables
let myVariable = "Hello, world!";
const myConstant = 42;
// data types
let myString = "Hello, world!"; // string
let myNumber = 42; // number
let myBoolean = true; // boolean
let myArray = [1, 2, 3]; // array
let myObject = { name: "John", age: 30 }; // object
let myNull = null; // null
let myUndefined = undefined; // undefined
Operators
Master arithmetic, assignment, comparison, and logical operators for efficient manipulation and comparison of values.
// arithmetic operators
let x = 10 + 5; // 15
let y = 10 - 5; // 5
let z = 10 * 5; // 50
let w = 10 / 5; // 2
// comparison operators
let a = 5 > 3; // true
let b = 5 >= 5; // true
let c = 5 < 3; // false
let d = 5 <= 5; // true
let e = 5 == "5"; // true
let f = 5 === "5"; // false
let g = 5 != "5"; // false
let h = 5 !== "5"; // true
// logical operators
let i = true && false; // false
let j = true || false; // true
let k = !true; // false
Conditional Statements
Uncover the art of crafting if statements, else clauses, and switch statements to guide program flow based on conditions.
if (condition) {
// code to be executed if the condition is true
} else if (anotherCondition) {
// code to be executed if the condition is false and anotherCondition is true
} else {
// code to be executed if all conditions are false
}
Loops
Navigate looping structures including for loops, while loops, and do-while loops to iterate through data efficiently.
// for loop
for (let i = 0; i < 10; i++) {
// code to be executed
}
// while loop
let i = 0;
while (i < 10) {
// code to be executed
i++;
}
// do-while loop
let i = 0;
do {
// code to be executed
i++;
} while (i < 10);
Functions
Grasp the intricacies of function creation, parameter passing, and the importance of return values for reusable code.
// function declaration
function myFunction(parameter1, parameter2) {
// code to be executed
return result;
}
// function expression
const myFunction = function(parameter1, parameter2) {
// code to be executed
return result;
}
// arrow function
const myFunction = (parameter1, parameter2) => {
// code to be executed
return result;
}
Objects and Classes
Dive into JavaScript’s object-oriented features, from creating objects to defining classes, constructors, and methods.
// object literal
const myObject = {
property1: "value1",
property2: "value2",
method: function() {
// code to be executed
}
};
// class declaration
class MyClass {
constructor(parameter1, parameter2) {
this.property1 = parameter1;
this.property2 = parameter2;
}
method() {
// code to be executed
}
}
// creating an instance of a class
const myInstance = new MyClass("value1", "value2");
This is just a small sample of what JavaScript can do. There are many more concepts and syntax to learn, but this should give you a good starting point. Know more HTML Cheat Sheet.
Our JavaScript cheat sheet is your essential companion for traversing the complexities of this versatile language. With snippets and explanations covering variables, operators, conditionals, loops, functions, and object-oriented concepts, you’ll swiftly find solutions to coding challenges. Whether you’re honing your skills or seeking quick references, this cheat sheet is your tool for success. Keep it bookmarked, print it out, or share it with fellow developers—it’s a bridge to mastering JavaScript’s capabilities with finesse and flair.