“Python offers a wide range of built-in string methods that can make working with strings easier and more efficient. Here are some useful string methods in Python that you should know: Useful String Method in Python.
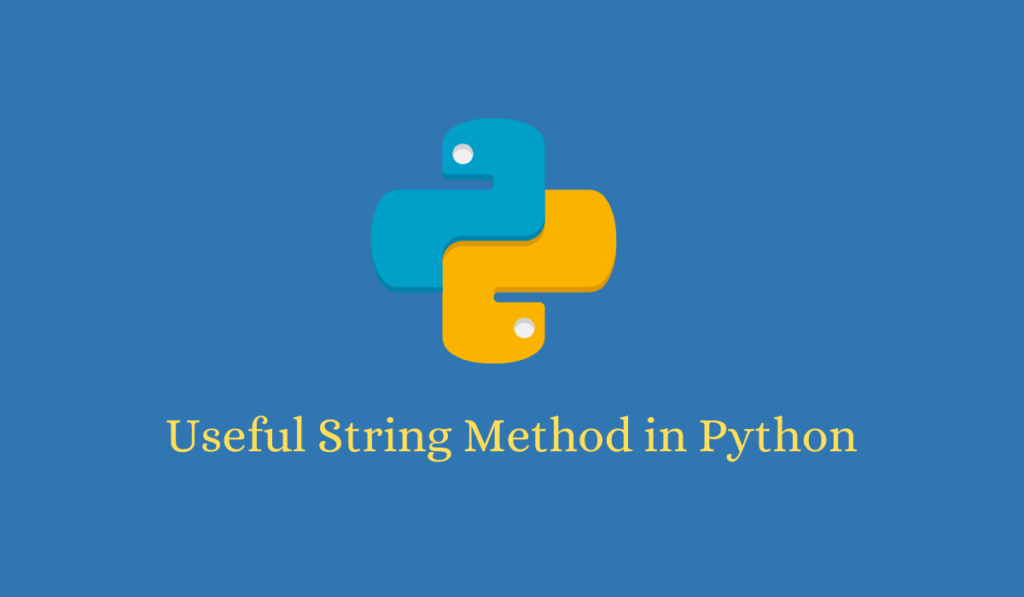
- strip(): The
strip()
method removes leading and trailing whitespace characters from a string. It’s incredibly useful for cleaning up user input or processing text files without unwanted spaces. For example:
string = " hello world "
string = string.strip()
print(string) # "hello world"
- replace(): The
replace()
method allows you to replace occurrences of a substring within a string with another substring. This method is handy for tasks like data cleaning, formatting, and string transformations. For example:
string = "Hello, World!"
string = string.replace("World", "Python")
print(string) # "Hello, Python!"
- split():The
split()
method splits a string into a list of substrings based on a specified delimiter. This method is crucial for breaking down textual data into manageable components, facilitating further analysis or processing. For example:
string = "apple,banana,orange"
fruits = string.split(",")
print(fruits) # ["apple","banana","orange"]
- join(): The
join()
method is the counterpart of thesplit()
method. It combines elements of an iterable, such as a list, into a single string using a specified delimiter. This method is essential for creating formatted output from structured data. For example:
fruits = ["apple","banana","orange"]
string = ",".join(fruits)
print(string) # "apple,banana,orange"
- find(): The
find()
method helps locate the index of a substring within a string. It’s useful for searching and extracting specific parts of text, enabling you to efficiently navigate and manipulate strings. For example:
string = "Hello, World!"
index = string.find("World")
print(index) # 7
These are just a few examples of the many useful string methods in Python. By mastering these methods, you can make working with strings easier and more efficient.Python’s versatile string methods empower developers to wield textual data with finesse. By mastering the strip()
, replace()
, split()
, join()
, and find()
methods, you unlock the ability to clean, transform, and manipulate strings for diverse programming tasks. These techniques not only streamline your code but also enhance your problem-solving capabilities. With these essential tools in your programming toolbox, you’re well-equipped to handle various text processing challenges effectively and efficiently.